Customize your PDP
The Product Detail Page (PDP) offers a versatile framework that allows you to modify its layout and functionalities to suit your specific business objectives. Below, you will find key areas where customization is possible, whether through our SCAYLE Panel or by editing the source code directly.
If you haven’t explored it yet, we suggest starting with our General Overview of the PDP to gain a better understanding of its features and layout options.
Product Details
To add, remove, or modify product information on the PDP, you need to update the corresponding attributes in the SCAYLE Panel. Detailed instructions can be found in the Attribute Groups section of the Panel Guide.
Each product attribute has a type
field that categorizes the product information, which in turn determines the product information accordion displayed on the PDP.
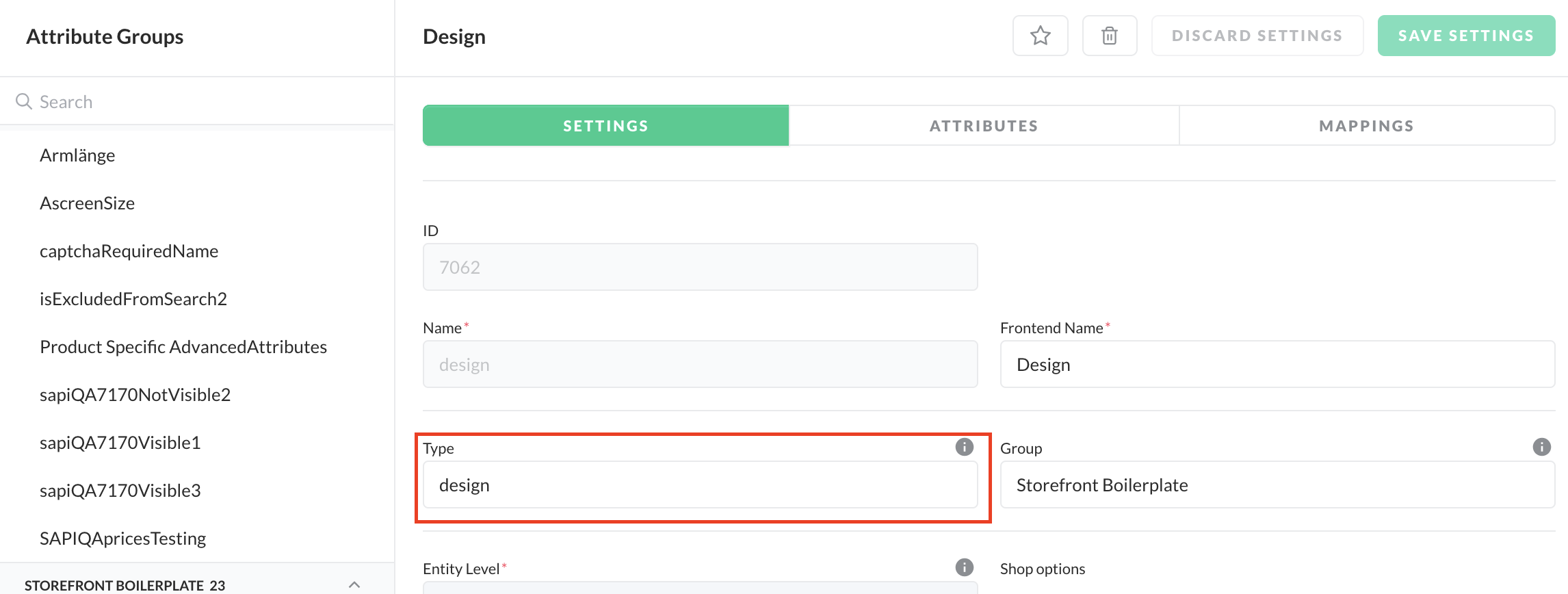
Add a type for the attribute to ensure it is displayed as product information on the PDP.
When setting or modifying a type
, it's important to make some adjustments in the Boilerplate code. Currently, the displayed types are limited to the following:
const types = ['fit_size', 'extras', 'design'];
If you require additional types or need to remove existing ones, simply update this array by adding or removing the corresponding type
string as defined in the Panel.
The final step involves creating an i18n key for the accordion title. This is handled dynamically, allowing the type
value to retrieve the appropriate translation text. The current i18n keys are located under pdp -> information
, with examples such as:
"fit_size": "Fit & Size",
"extras": "Extras",
"design": "Design"
By adding a new type
along with its related translation, an accordion with the corresponding title will automatically be generated on the PDP.
Recommendation Slider for Different Types of Related Products
The ProductRecommendation
component offers great flexibility when it comes to displaying recommendations. While it currently utilizes the combineWith
attribute to showcase products, this is just one of many possibilities. The component itself is designed to handle any list of product IDs you provide, meaning you could easily integrate personalized recommendations driven by a user's viewing history or past purchases.
Diverse Ways to Utilize the ProductRecommendation Component
- Recently Viewed Products: Enhance the user experience by displaying a list of recently viewed products. This can help users quickly revisit items they have shown interest in, improving the likelihood of conversion.
- Frequently Bought Together: Leverage the component to showcase products that are often purchased together with the currently viewed item. This can encourage additional purchases and enhance the average order value.
- Similar Products: Implement a feature that displays similar products based on attributes such as brand, category, or price range. This provides users with alternative options and helps them find products that meet their preferences.
- Personalized Recommendations: Integrate personalized recommendations based on the user's browsing history or past purchases. This tailored approach can increase engagement and drive conversions by presenting items that align with the user's interests.
- Seasonal Promotions: Highlight seasonal products or promotions using the slider. For example, during holiday seasons, showcase relevant gift items or discounts to encourage timely purchases.
- Trending Products or Best Sellers: Use the slider to highlight trending or popular products within a specific category. This feature can attract user attention to items that are currently in demand.
You might also want to filter out certain products from the recommendation slider (e.g. sold-out products). This is possible by applying any filter you might want to the products
list inside ProductRecomendations.vue
.
<template>
...
<template #default>
<template v-if="status === 'success'">
<ProductCard
v-for="(product, index) in availableProducts || []"
:key="product.id"
:product="product"
multiple-images
class="w-1/2 shrink-0 px-2 md:w-1/3 lg:w-1/4"
@click="trackProductCardClick(product, index)"
/>
</template>
</template>
...
</template>
<script setup lang="ts">
//...
const availableProducts = computed(() => {
return products.value?.filter((product) => !product.isSoldOut)
})
//...
</script>
Enabling Multiple Item Subscriptions
By default, ProductSubscription.vue
only adds a single item as a subscription, ignoring the quantity input. To support multiple items, you'll need to pass the quantity from ProductAction.vue
to ProductSubscription.vue
, then to ProductSubscriptionSelection.vue
, and finally to useSubscription.ts
.
Inside useSubscription
, reference the current quantity when creating the addToItem
:
const itemToAdd = computed<AddToBasketItem | undefined>(() => {
if (!selectedVariant.value) {
return
}
return {
variantId: selectedVariant.value?.id,
quantity: quantity.value, // <-- Use quantity instead of fixed value
customData: customData.value,
displayData: displayData.value,
productName:
getFirstAttributeValue(product.value.attributes, 'name')?.label || '',
interval: selectedInterval.value?.label,
}
})
Enhancing User Experience with the Product Detail Pop-up
The Product Detail Pop-up offers a seamless way for users to preview products and add them to their basket without disrupting their shopping experience or navigating away from the current page. Initially implemented for Free Gift products, this versatile pop-up can be easily integrated into other product cards throughout the application.
For instance, you can implement the pop-up for product cards within the Recommendation Slider, allowing users to quickly view product details and add items to their cart directly from the recommendations. Additionally, the pop-up can be utilized on product cards displayed on the Product Listing Page, enhancing user interaction and streamlining the purchasing process.
.png)
Product Card with an "Add to Basket" Button
Creating Low Stock Urgency
To let a user know that there are only a few items of a variant left, you can check the current stock of the variant using activeVariant.value?.stock.quantity
. Once the current stock is below a certain threshold and activeVariant.value?.stock.isSellableWithoutStock
is set to false
, you could display a hint on the PDP to create more urgency to buy the item.
.png)
Low Stock Disclaimer