Boosting your Add-ons visibility
Your Add-on is located in the Add-ons section. However, if your Add-on is used frequently, accessing it from this section may not be convenient enough.
To address this, we’ve provided two options to enhance the visibility of your Add-on:
- Adding Add-on to the SCAYLE Panel main navigation
- Adding Add-on to the SCAYLE Panel sidebar
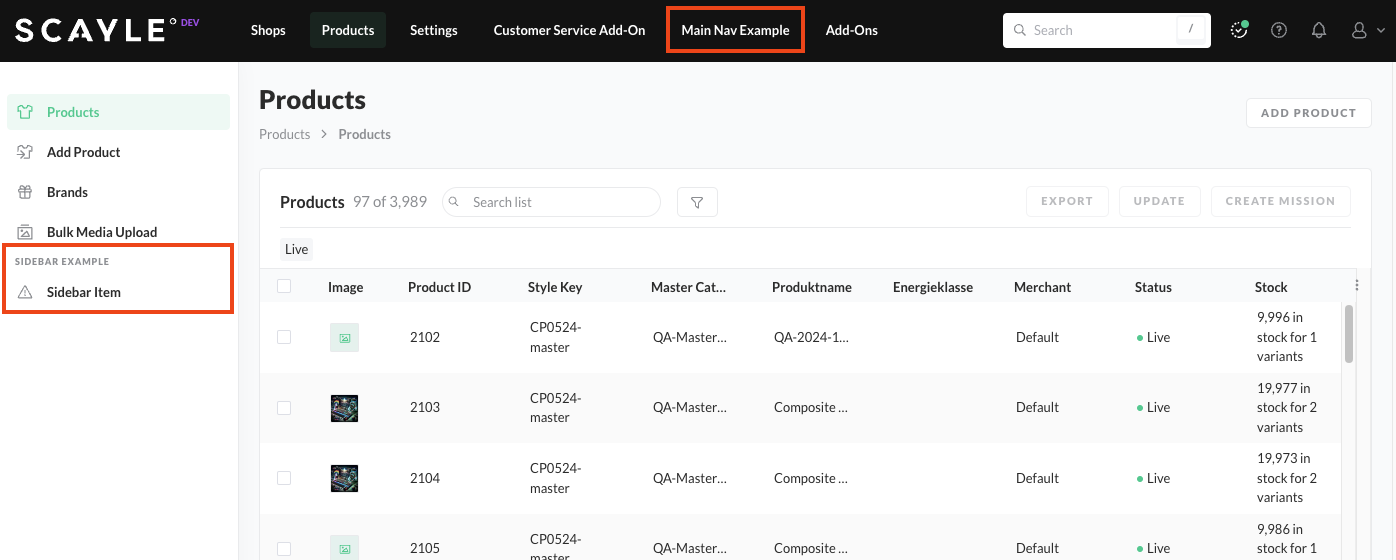
Possible positions of your Add-on
Adding Add-on to the SCAYLE Panel main navigation
To create your own entry in the main navigation you need to extend your Add-ons manifest.ts
...
const manifestRegistration = config(function (
registerApplication,
registerRoutes
) {
registerApplication({
name: ADD_ON_ID,
app: () => import("./add-on"),
});
// register routes
registerRoutes({
// Defining navigation item in the main navigation
main: [
{
id: "main-nav-item",
name: {
de: "Main Nav Example",
en: "Main Nav Example",
},
path: "foo/bar/baz",
location: {
position: "after",
id: "dashboard-show-in-main",
},
isAccessible(userData) {
// Decide if link should be shown based on the given userData
return true;
},
},
],
});
});
...
Adding Add-on to the SCAYLE Panel sidebar
Configuration to add your navigation item to the sidebar required modifying and creation various files:
Extend your manifest.ts
...
// defining nav items for the sidebar
const sidebarItems = [
{
name: {
de: "Sidebar Example",
en: "Sidebar Example",
},
group: "products::example-group",
isGroup: true,
},
{
id: "products-example-item",
icon: "warning",
name: {
de: "Sidebar Item",
en: "Sidebar Item",
},
group: "products::example-group",
path: "foo/bar",
sidebar: "products",
},
];
const manifestRegistration = config(function (
registerApplication,
registerRoutes
) {
registerApplication({
name: ADD_ON_ID,
app: () => import("./add-on"),
});
// register routes
registerRoutes({
// adding sidebar items to the products page sidebar
// available options are [shops, products, settings]
products: [...sidebarItems],
});
});
...
Add your route name
File: app/Support/RouteNames.php
Example: public const SHOP_ADDONS_OMNICHANNEL = 'shop.addons.omnichannel';
Add your navigation configuration
File: config/navigations.php
In this example, the navigation item is added to the shops
sidebar.
The route.name
is also used for checking permissions, so unless you want to create your own permission, use an existing and appropriate name that covers the desired permissions.
Example:
[...]
'shops' => [
[...],
[
'group' => 'navigation.group.channels',
'name' => 'navigation.' . RouteNames::SHOP_ADDONS_OMNICHANNEL,
'icon' => 'shirt',
'route' => [
'name' => RouteNames::SHOP_NAVIGATION_INDEX,
'routerIdentifier' => 'shop-addons-omnichannel',
'path' => '/shops/:shopId/addons/omnichannel',
'params' => [
'shop' => ':shopId',
],
'breadcrumb' => [
['name' => 'navigation.shop.index'],
['name' => 'navigation.' . RouteNames::SHOP_ADDONS_OMNICHANNEL],
],
],
],
[...]
]
Add translations
Files: lang/de/navigation.php
, lang/en/navigation.php
, …
Example: RouteNames::SHOP_ADDONS_OMNICHANNEL => 'Omnichannel Add-on',
Create and add your JS router file
Create your dedicated router file: resources/assets/application/router/routes/shop-omnichannel.js
Example:
export default [
{
path: 'addons/omnichannel',
name: 'addons-omnichannel',
component: () => import('@/pages/shop/omnichannel/Teaser.vue'),
meta: {
sidebar: 'shops',
breadcrumb: [
{ name: 'navigation.shop.index', route: { name: 'shop-list' } },
{ name: ':shopName', route: { name: 'shop-overview' } },
{ name: 'navigation.shop.addons' },
{ name: 'navigation.shop.addons.omnichannel' },
],
},
},
];
Add your router file to routes/index.js
File: resources/assets/application/router/routes/index.js
Example:
[...]
import shopOmnichannel from '@/router/routes/shop-omnichannel';
export default [
[...]
{
[...]
children: [
[...]
...shopOmnichannel,
]
},
];
Create your landing page
File: resources/assets/application/pages/shop/omnichannel/Teaser.vue
Example:
<template>
<div>
<h1>Hello World</h1>
</div>
</template>
<script setup lang="ts">
</script>
<style scoped lang="scss">
</style>