Composables
The product listing feature provides composables that provide logic for the core product listing features, such as filters, categories, sorting, and SEO data manipulation. Available through the corresponding NPM module @scayle/storefront-product-listing
these composables come with configurable parameters to help implement the feature in your project.
useFiltersForListing
A composable that provides filtering logic for an active category.
The composable is responsible for fetching the available filters and handling the application and reset functionalities. The process of applying filters varies depending on the filter typLogic
When a new filter is applied:
- The filter value is mapped with its key into a Record type.
- This mapped filter is then merged with the current query.
- The updated query is applied using
await router.push({ query: { ...newQuery } })
.
To reset a filter:
- The key of the filter to be reset is removed from the query.
- The updated query is then applied using
await router.push({ query: { ...newQuery } })
again.
By following this approach, we ensure that filters are managed efficiently and dynamically within the application.
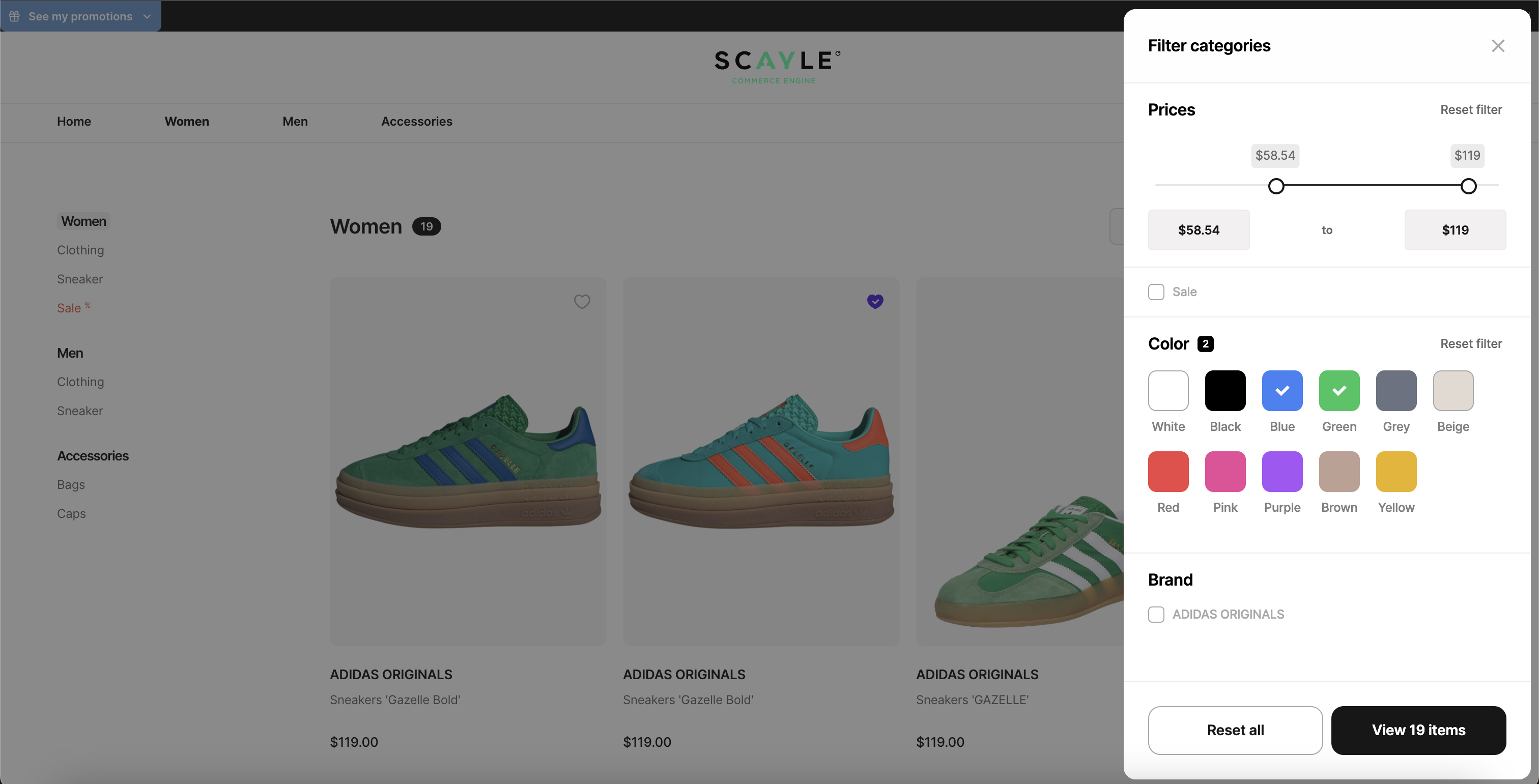
Desktop Filter Flyout
Parameters
options
(Optional) Configuration options for filtering products.
params
is to control product fetch behavior, excluding category
.
fetchingOptions
are options for fetch timing, such as lazy loading or immediate fetching.
fetchingKey
is a optional unique key to control the fetching cache.
Returns
UseFiltersForListingReturn
It returns extended filter data promise with specific properties. Those properties are:
refresh
: call for fetching the filters.error
: returns "useFilters" error.status
: returns "useFilters" status.availableFilters
: returns computed available filters.filteredProductCount
: returns filtered filters count.
useProductListSort
A composable that handles product list sorting.
Parameters
route
(Required) Route object to handle queries
options
(Optional) An options object that represents sorting behavior configuration. Available options are:
sortingOptions
- Array of available sorting options.defaultSortingKey
- The default sorting key will be applied if no specific sort is selected.
Default Behavior: An object with sortingOptions
and defaultSortingKey
default values
Returns
It returns computed properties and methods related to sorting. Those properties are:
selectedSort
- The currently selected sorting option.sortLinks
- Links with updated query parameters for each sorting option.isDefaultSortSelected
- Boolean indicating if the default sort is selected.
useProductsForListing
Composable to fetch and manage products by category with configurable options.
Parameters
options
(Optional) Configuration options for fetching products.
params
is to control product fetch behavior, excluding category
.
fetchingOptions
are options for fetch timing, such as lazy loading or immediate fetching.
fetchingKey
is a optional unique key to control the fetching cache.
Returns
products
A computed array of products in the specified category.
pagination
A computed object containing pagination details for the product list.
totalProductsCount
A computed total count of products in the category.
paginationOffset
A computed offset for the current page based on the pagination data.
status
A reactive boolean indicating if products are currently being fetched.
error
A reactive error object, null if no error occurred.
useProductListingSeoData
A composable function to generate SEO-related data for a product listing page.
Parameters
breadcrumbs
(Required) Breadcrumb items
route
(Required) Route object to handle queries
urlParams
(Required) URL data for constructing the canonical link.
Returns
title
A computed string that is constructed from breadcrumb values.
robots
A computed robots. Value can be "index,follow"
or "noindex,follow"
.
canonicalLink
A computed canonical link.
categoryBreadcrumbSchema
A computed breadcrumb schema.
useAppliedFilters
The useAppliedFilter
composable formats all active filters into a ProductSearchQuery
object. This object is essential for:
- Identifying which filters are currently applied.
- Fetching filtered filters and products based on the applied filters. Additionally,
useAppliedFilter
provides various computed values, such as the number of applied filters.
Parameters
route
(Required) Route object to handle queries
AppliedFilterOption.filterPrefix
The prefix used to identify filter-related query parameters.
Returns
appliedFilter
A computed property returning a ProductSearchQuery
object based on the route's query parameters.
appliedFiltersCount
A computed property that returns the total count of applied filters. Counts minPrice/maxPrice as one and counts each attribute filter separately.
appliedAttributeValues
A computed property that returns a record mapping attribute keys to arrays of numbers representing selected values for each attribute.
appliedBooleanValues
A computed property that returns a record mapping attribute keys to their boolean values for boolean filters.
areFiltersApplied
A computed property that returns true
if any filters are currently applied, otherwise false
.