Connect your ERP to SCAYLE
Stock updates
- OMS
- Tech
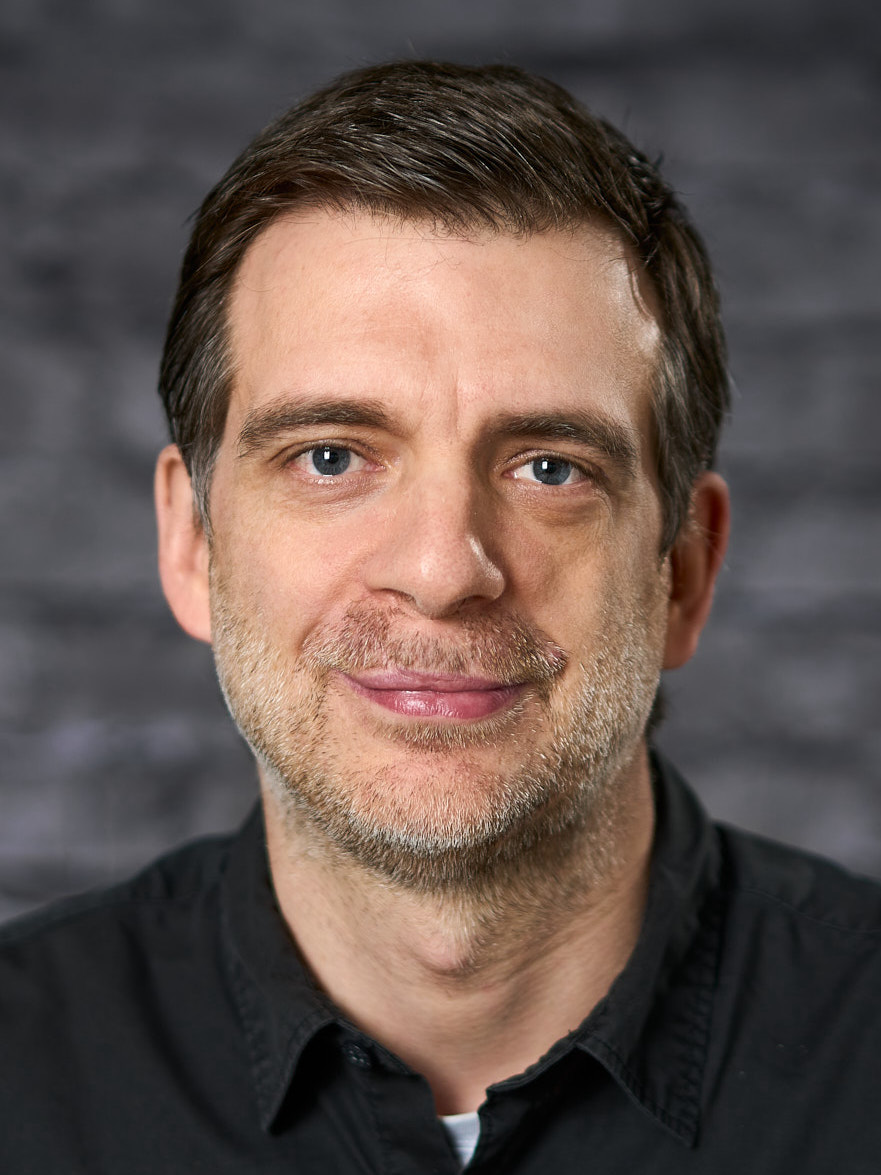
Birger Thoms
Lead Backend Developer
Stocks represent the number of the available items and are defined on the variant level. To ensure correct stock information, you can update the stock information with the actual stocks for the specific warehouse.
It is possible to sell variants infinitely by setting sellableWithoutStock
to true
when creating or updating a variant stock information. Available stock will be decreased as usual to zero, but the item can be still sold afterwards.
You can create stocks for a specific merchant by providing its reference key in the merchantReferenceKey
field.
On one side of the process, SCAYLE receives information about available stocks from various merchants. On the other side, when a customer places an order through the Checkout, the system ensures that the requested quantity of a product from a specific merchant is temporarily reserved in a designated warehouse. This reservation occurs silently without notifying the merchant.
The reservation process is triggered at the moment the payment provider confirms the payment or payment reservation. The actual order is then placed 60 seconds later, and if there are any errors, it attempts to retry for up to 2 days.
There are two ways to release quantity blockers:
Creating Stock Information: Quantity information can be updated via Admin API. The quantity blocker is only released when the stock updates timestamp is younger than the quantity blocker.
For more details, see Create Stock Information.
Order Cancellation via Checkout: When an order is cancelled via the Checkout, the system automatically adjusts the quantity by releasing the previously reserved amount.
Parameter | Details |
---|---|
quantity | Integer Current quantity of SKU. |
warehouseReferenceKey | String Reference key of warehouse for which the stock update is related to. |
changedAt | String Date time when the stock changed in ISO 8601 format. |
sellableWithoutStock | Boolean Defines if the variant can be sold even when the available stock is 0. |
merchantReferenceKey | String A merchant reference key the stock belongs to. |
Learn how to get multiple stocks.
This method can be used to get a collection of the latest product variant stock informations.
This method returns the current quantity for each warehouse.
When requesting stock, you can extend the query by specifying several product and variant identifier.
let response = await adminApi.apis.Stocks.getProductVariantStocks({variantIdentifier: variantIdentifier});
let stocks = response.body.entities;
Param Name | Type | Description |
---|---|---|
id | integer | ID assigned by SCAYLE. |
referenceKey | string | A key that uniquely identifies the variant of a product (usually an SKU) within the tenant's ecosystem. |
ean | string | An EAN that refers to a product variant. |
attributes | Attribute[] | A list of attributes attached to the product variant. |
prices | ProductVariantPrice[] | A list of prices attached to the product variant. |
stocks | ProductVariantStock[] | The product variant stock information |
customData | CustomData | |
isComposite | boolean | Indicates whether the variant is composite. |
relatedVariants | RelatedProductVariant[] | A list of variants that belong to the composite variant. |
let response = await adminApi.apis.Stocks.getProductVariantStocks({variantIdentifier: 1});
let stocks = response.body.entities;
stocks.forEach(
stock => console.log(stock.warehouseReferenceKey + ':' + stock.quantity)
);
You can update stock information by overriding existing information with a more recent change on warehouse stocks.
This method can be used to create a product variant stock change.
let response = await adminApi.apis.Stocks.createProductVariantStock(
{variantIdentifier: variantIdentifier},
{requestBody: newStock}
);
let createdStock = response.body;
If you provide a changedAt
date which is older than the last processed stock information, the stock will not change as this will be considered as outdated information. In this case the method will return the current stock. If you would like to find out if the information you sent was outdated, you have to compare what you’ve sent to what was returned in the response.
Sending a stock update without sellableWithoutStock
flag will not reset the value to false
(the default value), but keep the last stored value of this flag. If no value was previously set, the flag is set to the default value.
It is not possible to update stock information for composite product variants.
merchantReferenceKey
can be provided to create merchant-specific stocks. Note that merchantReferenceKey
must refer to one of the merchants provided on product creation.
If you send stocks by specifying a merchantReferenceKey
for which no product variant exists, you will receive the error message MERCHANT_REFERENCE_KEY_OUT_OF_RANGE
.
Make sure the provided warehouseReferenceKey
exists before you start creating stock.
Be aware that the referenced warehouse must be linked to a shop country. Otherwise, the shop country cannot use the stock.
parameter | Type | Required | Details |
---|---|---|---|
quantity | integer | true | Current quantity of SKU. |
warehouseReferenceKey | string | true | Reference key of warehouse for which the stock update is related to. |
changedAt | string | true | time when the stock changed in ISO 8601 format. |
sellableWithoutStock | boolean | ? | Defines if the variant can be sold even when the available stock is 0. |
merchantReferenceKey | string | ? | A merchant reference key the stock belongs to. |
let newStock = {
quantity: 10,
warehouseReferenceKey: "myWarehouse",
changedAt: "2020-07-23T11:30:58+00:00",
merchantReferenceKey: "merchant-1"
};
let response = await adminApi.apis.Stocks.createProductVariantStock(
{variantIdentifier: 1},
{requestBody: newStock}
);
let createdStock = response.body;
It is possible to set the sellable without stock flag for a given product variant without updating its quantity.
This method can be used to create or update the sellableWithoutStock
flag.
let response = await adminApi.apis.Stocks.setSellableWithoutStock(
{variantIdentifier: variantIdentifier},
{requestBody: sellableWithoutStock}
);
let sellableWithoutStock = response.body;
It is not possible to update stock information for composite product variants.
merchantReferenceKey
can be provided to set the sellableWithoutStock flag for merchant-specific variants. Note that merchantReferenceKey
must refer to one of the merchants provided on product creation.
If you try to set sellableWithoutStock flag by specifying a merchantReferenceKey
for which no product variant exists, you will receive the error message MERCHANT_REFERENCE_KEY_OUT_OF_RANGE
.
Make sure the provided warehouseReferenceKey
exists before you start creating stock.
Be aware that the referenced warehouse must be linked to a shop country. Otherwise, the shop country cannot use the stock.
If you try to set sellableWithoutStock flag by specifying a warehouseReferenceKey
that has no quantity defined yet, you will receive the error message QUANTITY_NOT_SET_FOR_WAREHOUSE .
parameter | Type | Required | Details |
---|---|---|---|
warehouseReferenceKey | string | true | Reference key of warehouse for which the sellableWithoutStock flag update is related to. |
sellableWithoutStock | boolean | true | Defines if the variant can be sold even when the available stock is 0. |
merchantReferenceKey | string | false | A merchant reference key the sellableWithoutStock flag belongs to. |
let newSellableWithoutStock = {
warehouseReferenceKey: "myWarehouse",
merchantReferenceKey: "merchant-1",
sellableWithoutStock: true
};
let response = await adminApi.apis.Stocks.setSellableWithoutStock(
{variantIdentifier: 1},
{requestBody: newSellableWithoutStock}
);
let sellableWithoutStock = response.body;
It's possible to create/delete stock quantity reservations via Admin API when orders are handled outside of SCAYLE.
This method allows you to create a new stock quantity reservation.
const response = await adminApi.apis.Reservations.create(
{},
{requestBody: reservations}
);
let createdStock = response.body;
parameter | Type | Required | Details |
---|---|---|---|
id readOnly | integer | true | Reservation ID. |
warehouseReferenceKey | string | true | Reference key of warehouse for which the stock update is related to. |
referenceKey | string | true | Reservation key, must be unique. |
variant | ReservationVariant | true | A merchant reference key the stock belongs to. |
error readOnly | ReservationError | false | Information of why the given reservation was not created. |
parameter | Type | Required | Details |
---|---|---|---|
id | integer | if referenceKey is not provided | Product variant ID. |
referenceKey | string | if id is not provided | Product variant's merchant identifier. |
merchantReferenceKey | string | false | Merchant key. If not provided, it's assumed that the given variant belongs to the default merchant. |
parameter | Type | Required | Details |
---|---|---|---|
errorKey | string | true | Error key. |
message | string | true | Error description. |
const reservations = [];
const reservation1 = {
referenceKey: 'order4711-item3',
warehouseReferenceKey: 'myWarehouse',
variant: {
id: 123,
merchantReferenceKey: 'merchant-1',
}
};
const reservation2 = {
referenceKey: 'order4711-item4',
warehouseReferenceKey: 'myWarehouse',
variant: {
referenceKey: 'foo-456789',
merchantReferenceKey: 'merchant-1',
}
};
reservations.push(reservation1);
reservations.push(reservation2);
const result = await client.apis.Reservations.createReservations({}, {requestBody: newProduct});
This method allows deleting a stock quantity reservation.
await adminApi.apis.Reservations.delete({reservationIdentifier: "key=my-key"});
client.apis.Reservations.deleteReservation({reservationIdentifier: "key=my-key"});
Connect your ERP to SCAYLE
Birger Thoms
Lead Backend Developer