Getting Started With SCAYLE
Add a Shop
- Getting started
- Tech
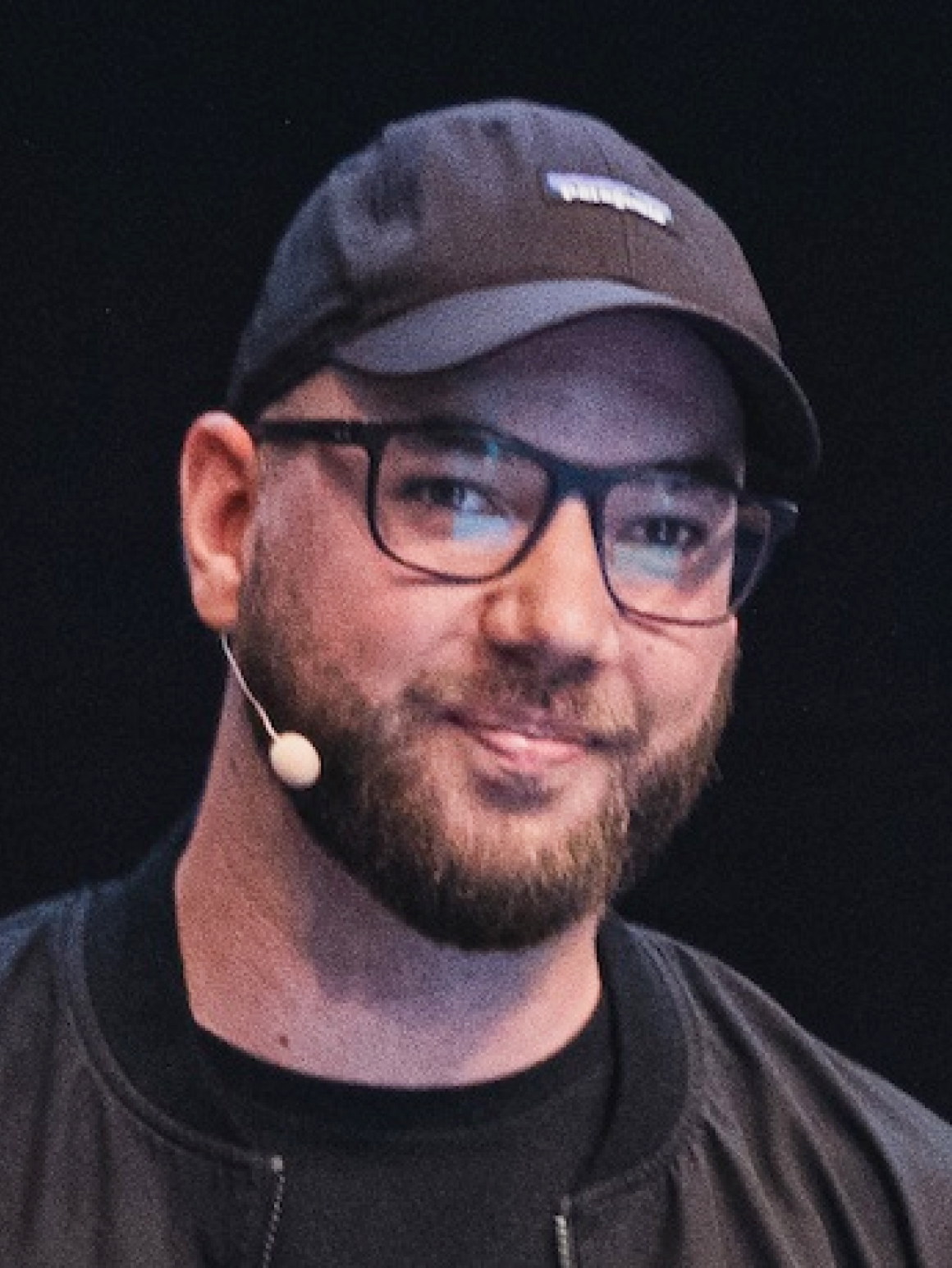
Robert Merten
VP Tech
The shop structure of SCAYLE consists of two levels:
Make sure to assign each shop to a country.
Shop settings can be overwritten at the country level.
For more information, check shop structure.
You can create your first minimal shop by defining the Name and a unique key for your instance:
let newShop = {
name: "My Shop",
key: "ms"
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: newShop});
let createdShop = response.body;
To be able to sell something you need to setup a Shop with a Shop Country. The Shop Country holds all the information (e.g. currency & language) for your new shop.
You can create a new Shop with a new Shop Country by defining the countries
information of the shop:
let newShop = {
name: "my Shop",
key: "ms",
logoUrl: "http://www.example.com/myLogo.png",
countries: [
{
countryCode: "DE",
defaultLanguageCode: "de_DE",
url: "http://www.example.com"
}
]
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: newShop});
let createdShop = response.body;
When you create a new Shop you can define a price group for the shop.
This should be used if you want to run multiple Shops (e.g. 'acme-fashion.com' and 'acme-shoes.com') and share the same prices in both shops. In this case you need to create both shops with the same price group key:
let fashionShopPayload = {
name: "ACME Fashion",
key: "fash",
logoUrl: "http://www.acme-fashion.com/myLogo.png",
priceGroupKey: "shared"
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: fashionShopPayload});
let fashionShop = response.body;
let shoeShopPayload = {
name: "ACME Shoes",
key: "shoe",
logoUrl: "http://www.acme-shoes.com/myLogo.png",
priceGroupKey: "shared"
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: shoeShopPayload});
let shoeShop = response.body;
In the example above the prices for your products will be shared for the same currencies. Sho both shops will have the same prices.
If you operate your Shop in multiple countries you can share the category tree across the Shop Countries by setting a shared Shop Category Tree ID.
This has the benefit that you don't need to manage your Shop Category Tree individually in every country:
let newShop = {
name: "My Shop",
key: "ms",
shopCategoryTreeId: 1
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: newShop});
let createdShop = response.body;
Typically you don't need to use this Feature.
If you just share your category tree across different Shop Countries the ID is set as default for you.
It's possible to use one SCAYLE instance to operate multiple shops which run under different legal entities.
If you want to create a new shop and assign the shop to a new legal entity you can simply add the legal entity ID to the create call:
let newShop = {
name: "My Shop",
key: "ms",
companyId: 1
};
let response = await adminApi.apis.Shops.createShop({}, {requestBody: newShop});
let createdShop = response.body;
To update your Shop you can lever the API / SDK in a very similar fashion than the Shop Create call.
It's not possible to update the `shopCategoryTreeId`, `companyId` and `priceGroupKey` settings after a Shop is created. If you want to change them you have to create a new Shop and delete the old one.
let response = await adminApi
.apis.Shops.getShop({shopKey: "ms"});
let shop = response.body;
shop.name = "My updated Shop";
let updateResponse = await adminApi.apis.Shops.updateShop({shopKey: shop.key}, {requestBody: shop});
let updatedShop = updateResponse.body;
If you want to start selling in a new Country you just need to create a new Shop Country for your Shop:
let newShopCountry = {
countryCode: "DE",
defaultLanguageCode: "de_DE",
supportedLanguageCodes: ["de_DE", "en_GB"],
url: "https://ms.de"
};
let response = await adminApi.apis.Shops.createShopCountry({shopKey: "ms"}, {requestBody: newShopCountry});
let createdShopCountry = response.body;
The Price Group is set on Shop level. If you create a new Shop Country the Price Group of the Shop is inherited.
You can update a Shop Country by the following call:
let response = await adminApi
.apis.Shops.getShopCountry({shopKey: "ms", countryCode: "DE"});
let shopCountry = response.body;
shopCountry.url = "https://ms.de";
let updateResponse = await adminApi.apis.Shops.updateShopCountry({shopKey: "ms", countryCode: "DE"}, {requestBody: shopCountry});
let updatedShopCountry = updateResponse.body;
You can request any given shop with its dependent countries.
This method can be used to get an existing shop by providing the shop key
.
This method allows to include nested resources using the with
parameter.
let response = await adminApi.apis.Shops.getShop({shopKey: shopKey});
let shop = response.body;
Shop read can be used with optional parameters - called options:
Parameter | Details |
---|---|
with | String Allows to load the following nested resources within this request:
|
See this example for details on how to use options.
let response = await adminApi.apis.Shops.getShop({shopKey: "ms"});
let shop = response.body;
console.log(shop.name);
let response = await adminApi.apis.Shops.getShop({shopKey: "ms", with: "countries.properties,countries.assortment"});
let shop = response.body;
shop.countries.forEach(
country => country.properties.forEach(
property => console.log(property.key + ": " + property.value)
)
);
SCAYLE allows you to request multiple shops at the same time considering different parameters to narrow down the query, e.g., price group key.
This method can be used to get a collection of existing shops. It is possible to refine the search by applying filters in the options.
This method allows to include nested resources using the with
parameter.
let response = await adminApi.apis.Shops.getShops();
let shops = response.body.entities;
Shop collection read can be used with optional parameters - called options:
Parameter | Details |
---|---|
with | String Allows to load the following nested resources within this request:
|
limit | Integer Maximum number of items in the result. (default: |
filters[priceGroupKey] | String Comma-separated list of price group keys. |
See this example for details on how to use options.
let response = await adminApi.apis.Shops.getShops();
let shops = response.body.entities;
shops.forEach(
shop => console.log(shop.name)
);
let response = await adminApi.apis.Shops.getShops({
"filters[priceGroupKey]": "MyPriceGroupKey"
});
let shops = response.body.entities;
shops.forEach(
shop => console.log(shop.name)
);
Shops and assortments can be entirely customized, but can also share specific information to facilitate multi-shop management within a single SCAYLE instance.
Some of the core functions of SCAYLE relate to the creation and administration of shops. The system allows individual settings for each shop within the instance. For example, for category trees and assortments. However, the system also lets shops inherit certain information from other shops, if needed.
Assortments of different shops can be customized based on:
The system enables users to assign different assortments and product scopes for each shop. They can define new assortments on a highly granular level, using inclusion and exclusion rules. All rules can be applied to:
In SCAYLE, users can define shop-specific category trees, which allows them to adapt quickly to changes and adjust product data for an individual shop. Similar to creating shop assortments, inclusion and exclusion rules define which products should be included in which shop category. All rules can be created for each category level and will automatically be inherited by the respective subcategories.
There are different options available for each shop category:
It is possible to manage several shops in the same instance of the SCAYLE Panel. Every (new) shop can run entirely independently from any other shop. Some everyday use cases for operating multiple shops are:
Since we highly value and support our client's flexibility, there are no limitations on how shops can be handled in the same instance. It is possible to operate shops with overlapping assortments and shared products. Each shop will run independently from any other shop that is part of the same instance.
Additional product information can be added on the shop level to the predefined, global product structure. Similar to the master data configuration, users can work with different data types.
Information related to shop-specific attributes is connected to the Storefront API and will only be shown on the product detail page if a customer is visiting this specific shop.
With this method, it is possible to get the current shop configuration with all shop-specific properties. It is possible to modify these properties through the SCAYLE Panel.
All examples require a configured Storefront API client to be available as client
.
See Authentication for how to set it up.
const response = await client.shopConfiguration.get();
{
"shopId": 1001,
"name": "SCAYLE Demo",
"shopCustomData": {},
"properties": [],
"customData": {},
"country": "DE"
}
Getting Started With SCAYLE
Robert Merten
VP Tech