Getting Started With SCAYLE
Create and attach a warehouse
- Getting started
- Tech
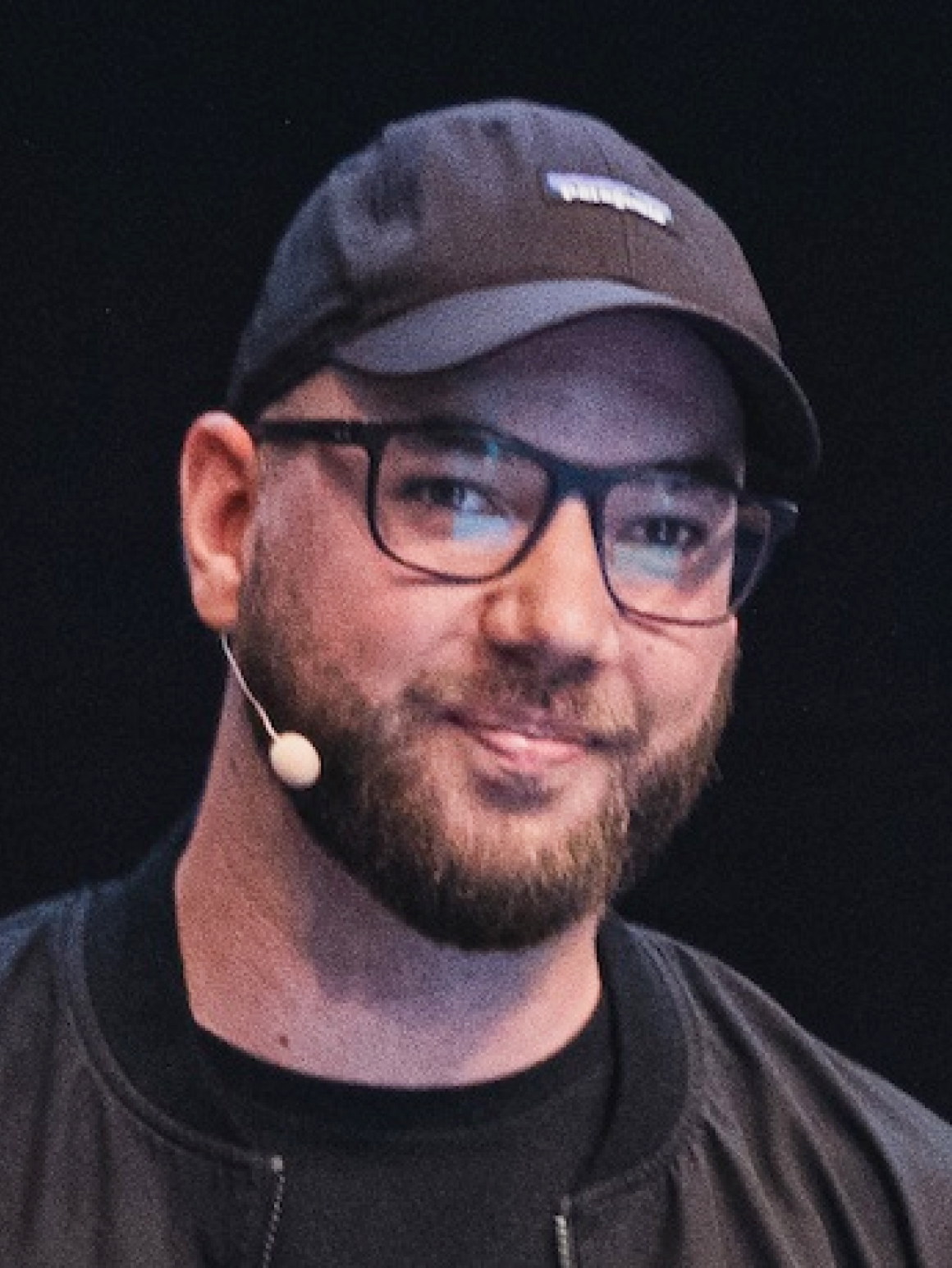
Robert Merten
VP Tech
SCAYLE comes with multi-warehouse support, allowing you to assign warehouses to merchants and shop countries.
A shop country warehouse represents a warehouse assigned to a shop country.
When assigning a warehouse to a shop country, you can define a priority and a package group.
Shop country warehouses can have a priority
, which allows defining from which warehouse an item should get shipped first. The higher the value, the higher the priority.
See Attach Group Overview for more information.
Warehouses must be configured before sending stock information as variants.
Remember, variants are the last level within SCAYLEs product structure and represent the sellable entity. See Product Structure.
In the next sections, we’ll explain how our warehouses work, how many you might need, and how to configure them based on our example scenario.
In the world of SCAYLE, a warehouse is a logical entity that holds stock for specific variants. Every warehouse is assigned to one or more shop countries.
Need a refresher on shop countries? Check: What is a “Shop Country” in SCAYLE?
A warehouse can represent a physical warehouse, but it doesn’t have to.
Furthermore, warehouses outline priority that defines which stock should be sold first per variant. You can configure priority on the shop country level. The higher the value, the higher the priority. Also, stock assigned to a specific warehouse can have different delivery times on the shop country level (please refer to Delivery Estimations for more information about this feature.)
Warehouses can be configured using the Admin API. However, in most cases, you will only configure warehouses once without further changes, so we recommend using the SCAYLE Panel.
The following set of questions can help you to identify which warehouse structure you need:
Now let’s see how we would set up warehouses for our Fashion Store. Our example operates 2 warehouses. Here are some additional details we need to configure our warehouses:
The following diagram illustrates the scenario:
Warehouses
In this situation, we need to create two warehouses. The warehouse in Frankfurt will be assigned to all shop countries, while the warehouse in Basel will only be assigned to the 2 shop countries of Switzerland. (We identified the need to have three shop countries in this chapter: Shop Structure).
Settings > Merchant management > Select default merchant > Edit
.Add Warehouse.
Shops > Shop Name > Shop Country > Storefront > Warehouses
.Storefront - Add Warehouse.
Great job! Now you’ve learned the warehouse basics and assigned them to our Fashion Store. Time to send stock information in the next section.
It is not possible to modify warehouse's referenceKey
after its creation!
This method is used to create a new warehouse on a global level.
let response = await adminApi.apis.Warehouses.createWarehouse({}, {requestBody: warehouse});
warehouse = response.body;
Parameter | Type | Description | Required |
---|---|---|---|
id | integer | The ID of the warehouse created by SCAYLE. | true |
referenceKey | string | A key that uniquely identifies the warehouse within the tenant's ecosystem. | true |
merchants | Merchant | A list of merchants the warehouse is attached to. | false |
shopCountries | WarehouseShopCountry | Shop country information related to the warehouse. | false |
let newWarehouse = {
referenceKey: "my-warehouse"
};
let response = await adminApi.apis.Warehouses.createWarehouse({}, {requestBody: newWarehouse});
let createdWarehouse = response.body;
console.log(createdWarehouse.id);
Learn how to attach a warehouse to a shop country with its priority and package group.
When you attach a warehouse to a shop country, you can set a priority and assign it to a package group.
This method is used to attach a warehouse to a shop country as described here.
referenceKey
cannot be modified.let response = await adminApi.apis.Warehouses.createShopCountryWarehouse(
{ shopKey: shopKey, countryCode: countryCode },
{ requestBody: shopCountryWarehouse }
);
let createdShopCountryWarehouse = response.body;
Parameter | Type | Description | Required |
---|---|---|---|
id | integer | The ID of the warehouse created by SCAYLE. | true |
referenceKey | string | A key that uniquely identifies the warehouse within the tenant's ecosystem. | true |
priority | integer | The priority of the warehouse. The higher the value, the higher the priority. | false |
packageGroup | PackageGroup | The assigned package group of the warehouse. | false |
let newShopCountryWarehouse = {
referenceKey: "my-warehouse",
priority: 100
};
let response = await adminApi.apis.Warehouses.createShopCountryWarehouse(
{
shopKey: "ms",
countryCode: "DE"
},
{
requestBody: newShopCountryWarehouse
}
);
let createdShopCountryWarehouse = response.body;
Create a shop warehouse with package group:
let newShopCountryWarehouse = {
referenceKey: "my-warehouse",
packageGroup: {
id: 3
}
};
let response = await adminApi.apis.Warehouses.createShopCountryWarehouse(
{
shopKey: "ms",
countryCode: "DE"
},
{
requestBody: newShopCountryWarehouse
}
);
let createdShopCountryWarehouse = response.body;
This method is used to attach an existing warehouse to a merchant.
await adminApi.apis.Warehouses.attachMerchantWarehouse({merchantIdentifier: 1, warehouseIdentifier: 1});
Attaching the same warehouse to the merchant twice will result in 400 response code with MERCHANT_WAREHOUSE_ALREADY_EXISTS
error key.
await adminApi.apis.Warehouses.attachMerchantWarehouse({merchantIdentifier: 1, warehouseIdentifier: 1});
This method is used to detach a warehouse from a merchant.
await adminApi.apis.Warehouses.detachMerchantWarehouse({merchantIdentifier: merchantIdentifier, warehouseIdentifier: warehouseIdentifier});
await adminApi.apis.Warehouses.detachMerchantWarehouse({merchantIdentifier: merchantIdentifier, warehouseIdentifier: warehouseIdentifier});
You can request multiple warehouses along with the related data such as merchants and shop countries.
This method is used to get a collection of existing warehouses.
Parameter | Type | Description | Required |
---|---|---|---|
with | string | Allows to load the following nested resources within this request: merchants, shopCountries | false |
limit | integer | Maximum number of items in the result. (default: 100 , maximum: 1000 ) | false |
filters[id] | string | Comma-separated list of warehouse IDs that should be used for filtering. | false |
filters[minId] | integer | Minimum warehouse ID of entities that should be returned. | false |
filters[maxId] | integer | Maximum warehouse ID of entities that should be returned. | false |
sort | string | Sort by the provided column. Available values: id. | false |
sortDir | string | Defines the sorting order: ascending or descending. Available values: asc (default), desc. | false |
let response = await adminApi.apis.Warehouses.getWarehouses();
let warehouses = response.body.entities;
You can request warehouses attached to a merchant using its identifier.
let response = await adminApi.apis.Warehouses.getMerchantWarehouses({merchantIdentifier: merchantIdentifier});
let warehouses = response.body.entities;
Parameter | Details |
---|---|
with | String Allows to load the following nested resources within this request:
|
limit | Integer Maximum number of items in the result. (default: |
filters[id] | String Comma-separated list of warehouse IDs that should be used for filtering. |
filters[minId] | Integer Minimum warehouse ID of entities that should be returned. |
filters[maxId] | Integer Maximum warehouse ID of entities that should be returned. |
sort | String Sort by the provided column. Available values: id. |
sortDir | String Defines the sorting order: ascending or descending. |
Parameter | Type | Description | Required |
---|---|---|---|
entities | Warehouse | A collection of warehouses. | true |
cursor | Cursor | An object containing information for pagination. | true |
let response = await adminApi.apis.Warehouses.getMerchantWarehouses({merchantIdentifier: 1});
let warehouses = response.body.entities;
warehouses.forEach(
warehouse => console.log(warehouse.id)
);
In SCAYLE, you can update shop country warehouse relation at any time. The provided information will replace current values and delete old ones.
This method is used to update an existing shop country warehouse relation.
referenceKey
cannot be modified.let response = await adminApi.apis.Warehouses.updateShopCountryWarehouse(
{ shopKey: shopKey, countryCode: countryCode, shopCountryWarehouseIdentifier: shopCountryWarehouseIdentifier },
{ requestBody: shopCountryWarehouse }
);
let createdShopCountryWarehouse = response.body;
let shopCountryWarehouse = {
referenceKey: "my-warehouse",
priority: 101
};
let response = await adminApi.apis.Warehouses.updateShopCountryWarehouse(
{
shopKey: "ms",
countryCode: "DE",
shopCountryWarehouseIdentifier: 1
},
{
requestBody: shopCountryWarehouse
}
);
let updatedShopCountryWarehouse = response.body;
Parameter | Type | Description | Required |
---|---|---|---|
with | string | Allows to load the following nested resources within this request: merchants , shopCountries | false |
limit | integer | Maximum number of items in the result. (default: 100 , maximum: 1000 ) | false |
filters[id] | string | Comma-separated list of warehouse IDs that should be used for filtering. | false |
filters[minId] | integer | Minimum warehouse ID of entities that should be returned. | false |
filters[maxId] | integer | Maximum warehouse ID of entities that should be returned. | false |
sort | string | Sort by the provided column. Available values: id. | false |
sortDir | string | Defines the sorting order: ascending or descending. Available values: asc (default), desc. | false |
Learn how to detach a warehouse from a shop country.
In SCAYLE, you can detach a warehouse from a shop country. Please make sure to consider all dependencies in advance.
This method can be used to detach a warehouse from a shop country.
This action can not be undone!
adminApi.apis.Warehouses.deleteShopCountryWarehouse(
{ shopKey: shopKey, countryCode: countryCode, shopWarehouseIdentifier: shopWarehouseIdentifier }
);
adminApi.apis.Warehouses.deleteShopCountryWarehouse(
{
shopKey: "ms",
countryCode: "DE",
shopWarehouseIdentifier: "key=my-warehouse"
}
);
This method is used to delete an existing warehouse.
SCAYLE allows you to delete a warehouse as long as it doesn't have any stock.
await adminApi.apis.Warehouses.deleteWarehouse({warehouseIdentifier: warehouseIdentifier});
Parameter | Details |
---|---|
id | Integer The ID of the warehouse created by SCAYLE. |
referenceKey | String A key that uniquely identifies the warehouse within the tenant's ecosystem. |
merchants | A list of merchants the warehouse is attached to. |
shopCountries | Shop country information related to the warehouse. |
await adminApi.apis.Warehouses.deleteWarehouse({warehouseIdentifier: 1});
Getting Started With SCAYLE
Robert Merten
VP Tech